What is it?
Code coverage, it is this magical number that will never hit 100% for a larger project, but near 100% should be doable right?
One of my projects is setup using the Clean Architecture and has a separate front end and back end test project.
For this article I will focus on the back end, written in C# with ASP.NET Core 3.1 using Xunit and FluentAssertions.
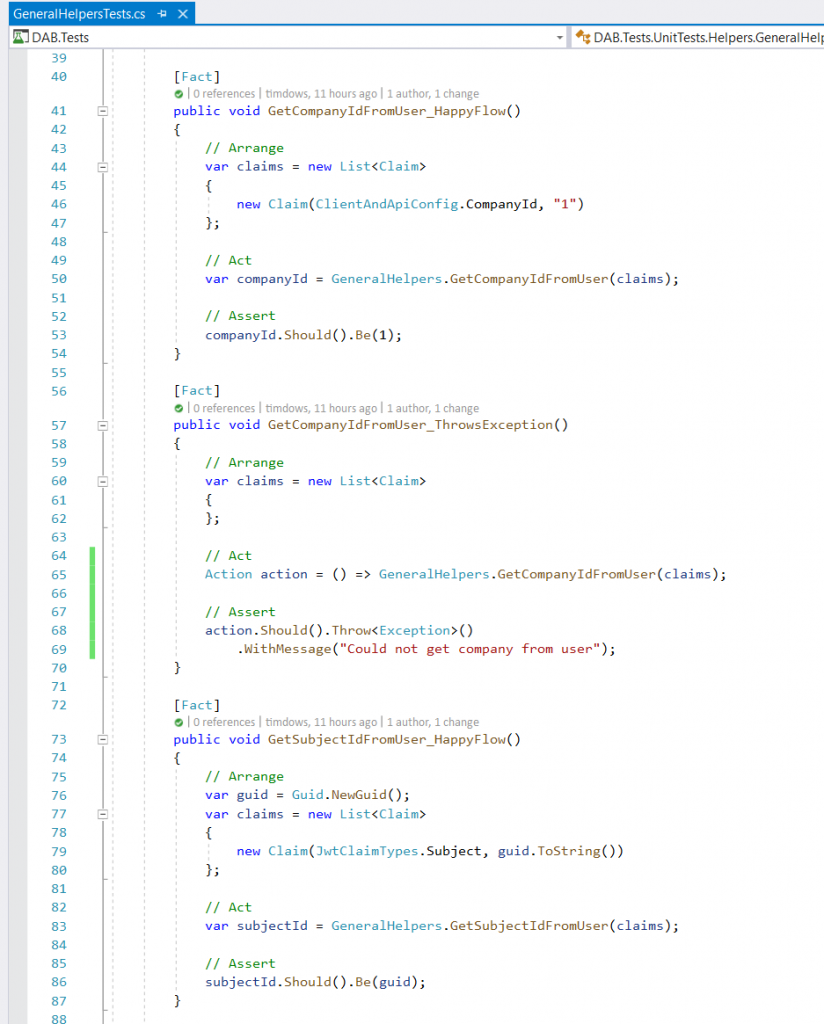
The challenge
Writing tests like above is straightforward, but a mistake is easaly made.
What mistake you ask?
Well, the one of missing a test implementation of one of the helper methods in my helper class.
As you can see, they have some good naming, but those might look a lot like each other, e.g.:
GetCompanyIdFromUser
GetIdentityServerIdFromUser
GetSubjectIdFromUser
They all start with Get
and end with IdFromUser
.
In my test class I implement them from top to bottom, like in the example image above in this post
GetCompanyIdFromUser_HappyFlow
GetCompanyIdFromUser_ThrowsException
GetSubjectIdFromUser_HappyFlow
GetSubjectIdFromUser_ThrowsException
And there it is, the sneaky mistake, the tests for GetIdentityServerIdFromUser
are missing.
Identity the missing tests using tools
Some options to identify are widely available
- Visual Studio Live Unit Testing, only for the Enterprise edition
- dotCover from Jetbrains
- Coveralls
- Coverlet
- altcover
But all of them at the moment of writing didn’t show me in a simple way that I was missing to test on of my helper methods.
I based my solution on a blog post on medium from Krishna Mohan: https://medium.com/swlh/generating-code-coverage-reports-in-dotnet-core-bb0c8cca66
It uses the following steps:
- Run dotnet test with a collector
- Change the coverage report to a xml version
- Generate a html report
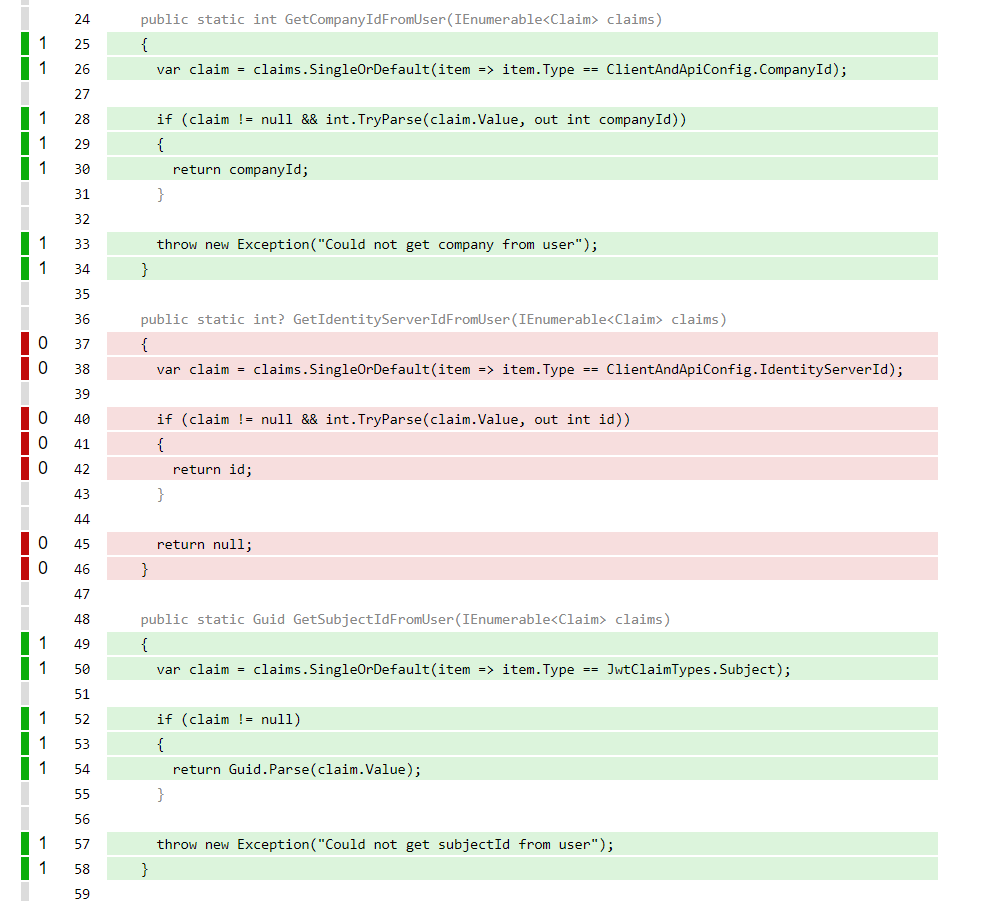
GetIdentityServerIdFromUser
Automate this report as far as possible
I created a powershell script that does exactely this.
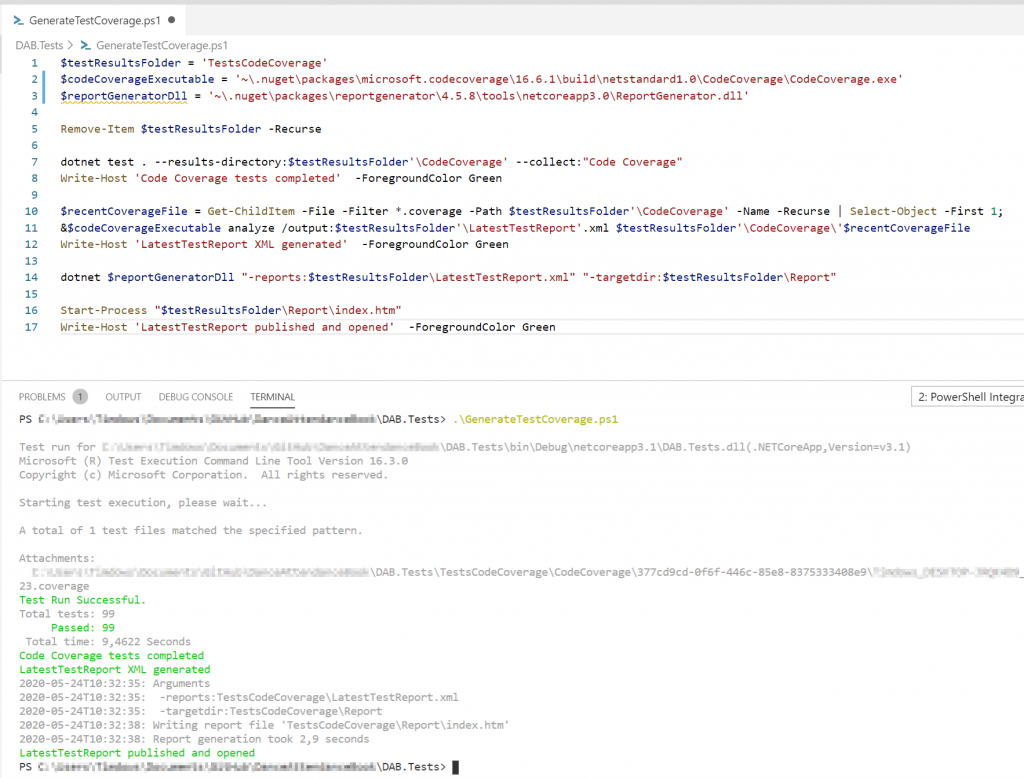
How to use it
1. Goto the test project and install the dependencies:
Install-Package Microsoft.CodeCoverage -Version 16.6.1
Install-Package ReportGenerator -Version 4.5.8
2. Place the GenerateTestCoverage powershell script in the test project directory
3. Run it 😀
Result
Some tweaking needs to be done as I will not test migrations with almost 100k lines of code, but is shows that for my core project, I am only just above a quarter, that needs to be improved!
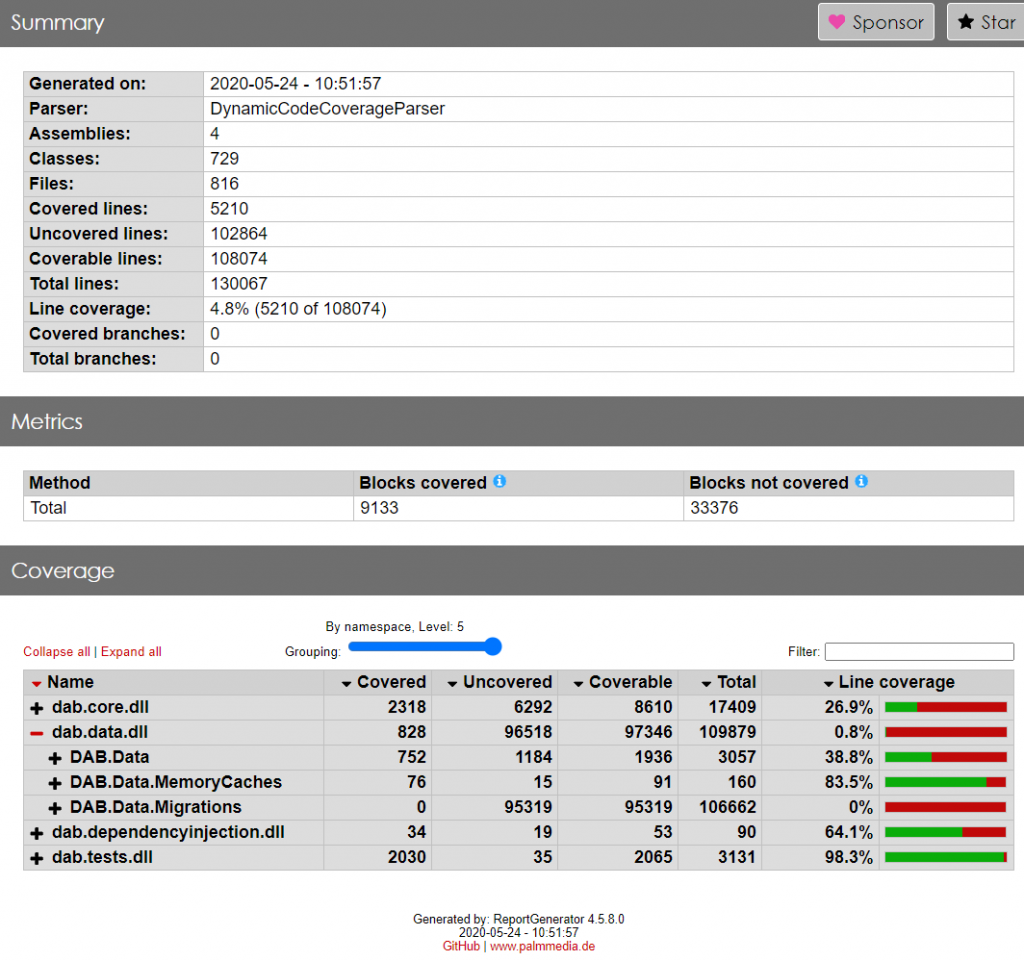
Great stuff! Can I also use the code coverage output in an Azure Devops dashboard or something?
It can for sure be added to a Azure DevOps build Pipeline. The results will than be in the aftifect directory.
Should be doable to add those to the dashboard