What is it? 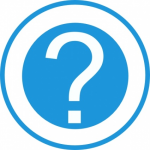
My objective consists of two parts, load the Angular2 template while it is being served by Razor and also apply lazy loading of the template itself.
I followed the post from Victor Savkin on https://vsavkin.com/angular-router-declarative-lazy-loading-7071d1f203ee
Lazy loading speeds up our application load time by splitting it into multiple bundles, and loading them on demand.
ASP.NET Core and Angular2 code 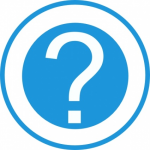
All the code can be found on my github page: https://github.com/timdows/webpages/tree/master/Angular2RazorViews
Load Angular2 template that is served by Razor
I took a random existing Angular2 project that I used before. It already serves the Angular2 application via ASP.NET Core 1.0.
In the Component section I changed template for templateUrl. This technique is called Component-Relative Paths.
This resulted changing the following files:
angular2App/app/project/project.component.ts
[code lang=”js”]
import { Component, OnInit } from "@angular/core";
@Component({
selector: "projectcomponent",
templateUrl: "view/projects/index.html"
})
export class ProjectComponent implements OnInit {
message: string;
constructor() {
}
ngOnInit() {
this.message = "From the angular2 ProjectComponent class";
}
}
[/code]
Controllers/Projects/ProjectsController.cs
[code lang=”csharp”]
public class ProjectsController : Controller
{
public IActionResult Index()
{
ViewData["Message"] = "Created at the ProjectsController";
return View();
}
}
[/code]
Views/Projects/Index.cshtml
[code lang=”csharp”]
<div>
@ViewData["Message"]<br/>
Some cshtml file generated data<br/>
{{message}}
</div>
[/code]
In order to get to the controller via a view route, I added a route in the app.UseMVC section
Startup.cs
[code lang=”csharp”]
app.UseMvc(routes =>
{
routes.MapRoute(
"viewRoute",
"view/{controller}/{action}.html",
new {controller = "Home", action = "Index"});
});
[/code]
The result is a complete Angular2 application with a view that has ViewBag data, normal cshtml data and an Angular2 message.
Lazy loading
As seen in the previous part, the file is loaded regardless if it is needed or not. Some documentation that I’m using to get it hopefully working in a couple of days.
https://vsavkin.com/angular-router-declarative-lazy-loading-7071d1f203ee
https://github.com/Quramy/angular2-load-children-loader
[2016-11-07]
https://devblog.dymel.pl/2016/10/06/lazy-loading-angular2-modules
http://gbataille.github.io/2016/02/16/Angular2-Webpack-AsyncRoute.html
https://medium.com/@daviddentoom/angular-2-lazy-loading-with-webpack-d25fe71c29c1#.qtv89zjhn
Hi, Tim!
Good article, thanks a lot.
Did you found how to load separate bundles by need?
I did not get lazy loading to work yet (I must also admit that I’ve only looked at it for half an hour). Hopefully it will be easier in the future.
Are you using WebPack or SystemJS to translate the assets into TypeScript? I have an Angular2 .NET Core project that uses WebPack and Angular isn’t able to fetch the views from the MVC controller.
I use WebPack for this, today I use all the bundled Angular-cli (package called @angular/cli today).
Make sure you use templateUrl and not template in your typescript component!
Hi there, excellent post! I’ve got a question.
How is the deal with the communication between the Model that you receive from the .net controller and the typescript component?
For example, I am returning a view and I’ve some fields filled and built with razor syntax: TextBoxFor, EditorFor, etc, but what I need is to assign those values that are in those fields, to their specifically properties in the typescript component.
@Html.TextBox(“nameTest”, null, new Dictionary() { { “[(ngModel)]”, “name” } }) where “name” is a prop in ts component.
That way I am having just component => template binding. What I need is the opposite, values set in the cshtml when the page is rendered, bind those values to the component.
I hope this is clear and hoping you can help me.
Thanks!