I’ve got a cronjob running to sync the images from my Raspberry Pi Timelapse project.
The cronjob does nothing more than calling rsync.
[code lang=”bash”]#!/bin/sh
if [ "$(pidof rsync)" ]
then
echo "process already running"
else
rsync –remove-source-files -chavzP –stats [email protected]:/home/pi/capture/ /volume1/projects/VWP\ Timelapse/timelapse\ construction
fi[/code]
Now I want to export information regarding the amount of pictures that are on the Synology.
For this I created a PHP script that, gave the http user access to read the projects share and made some open_basedir changes.
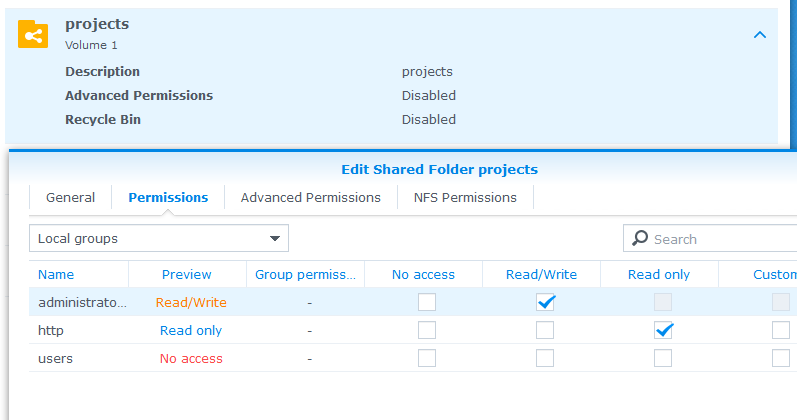
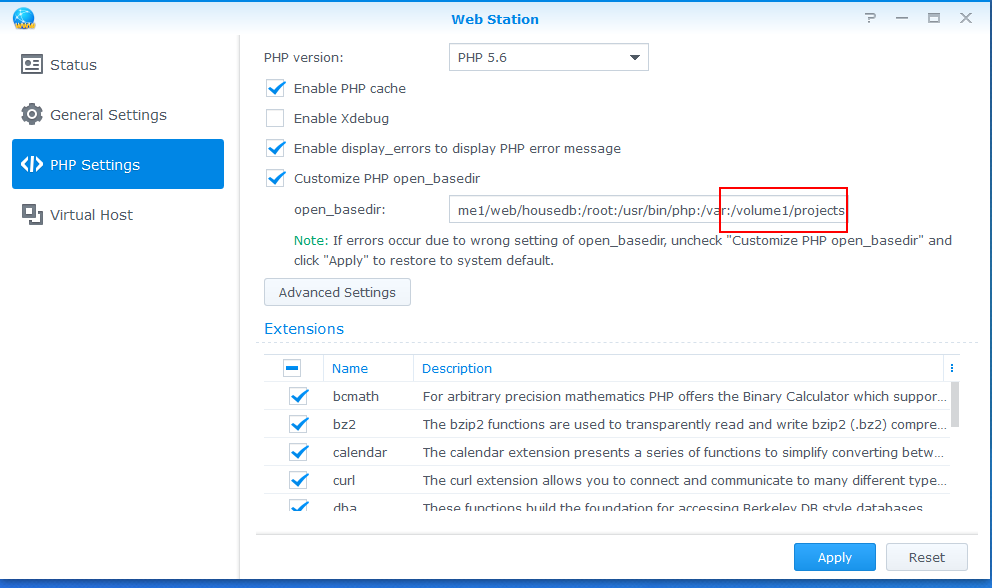
[code lang=”php”]<?php
// Allow from any origin
if (isset($_SERVER[‘HTTP_ORIGIN’])) {
// Decide if the origin in $_SERVER[‘HTTP_ORIGIN’] is one
// you want to allow, and if so:
header("Access-Control-Allow-Origin: {$_SERVER[‘HTTP_ORIGIN’]}");
header(‘Access-Control-Allow-Credentials: true’);
header(‘Access-Control-Max-Age: 86400’); // cache for 1 day
}
// Access-Control headers are received during OPTIONS requests
if ($_SERVER[‘REQUEST_METHOD’] == ‘OPTIONS’) {
if (isset($_SERVER[‘HTTP_ACCESS_CONTROL_REQUEST_METHOD’]))
// may also be using PUT, PATCH, HEAD etc
header("Access-Control-Allow-Methods: GET, POST, OPTIONS");
if (isset($_SERVER[‘HTTP_ACCESS_CONTROL_REQUEST_HEADERS’]))
header("Access-Control-Allow-Headers: {$_SERVER[‘HTTP_ACCESS_CONTROL_REQUEST_HEADERS’]}");
exit(0);
}
function formatSizeUnits($bytes) {
if ($bytes >= 1073741824) {
$bytes = number_format($bytes / 1073741824, 2) . ‘ GB’;
}
elseif ($bytes >= 1048576) {
$bytes = number_format($bytes / 1048576, 2) . ‘ MB’;
}
elseif ($bytes >= 1024) {
$bytes = number_format($bytes / 1024, 2) . ‘ kB’;
}
elseif ($bytes > 1) {
$bytes = $bytes . ‘ bytes’;
}
elseif ($bytes == 1){
$bytes = $bytes . ‘ byte’;
}
else {
$bytes = ‘0 bytes’;
}
return $bytes;
}
function folderSize ($dir) {
$size = 0;
foreach (glob(rtrim($dir, ‘/’).’/*’, GLOB_NOSORT) as $each) {
$size += is_file($each) ? filesize($each) : folderSize($each);
}
return $size;
}
function folderSizeFast ($dir) {
$output = shell_exec("du -hb ‘" . $dir . "’ | cut -f1");
$output = str_replace("\n", "", $output);
return $output;
}
header(‘Content-Type: application/json’);
if (isset($_GET[‘getexport’])) {
// open the file in a binary mode
$name = "export.json";
$fp = fopen($name, ‘rb’);
// send the right headers
header("Content-Length: " . filesize($name));
// dump the picture and stop the script
fpassthru($fp);
exit;
}
$path = ‘/xyz/’;
$retVal = new stdClass();
$retVal->path = $path;
$retVal->date = date("y-m-d H:i:s");
$dataArray = [];
$dir = new DirectoryIterator($path);
foreach ($dir as $fileinfo) {
if ($fileinfo->isDir() && !$fileinfo->isDot()) {
$fi = new FilesystemIterator($fileinfo->getRealpath(), FilesystemIterator::SKIP_DOTS);
$object = new stdClass();
$object->dirname = $fileinfo->getFilename();
$object->filecount = iterator_count($fi);
if(isset($_GET["forexport"])){
$object->size = folderSizeFast($fileinfo->getRealpath());
$object->formattedSize = formatSizeUnits($object->size);
}
array_push($dataArray, $object);
}
}
$retVal->files = $dataArray;
$json = json_encode($retVal);
if(isset($_GET["forexport"])){
file_put_contents("export.json", $json);
}
echo $json;
?>[/code]
Make sure that the export.json file exists and has appropriate permissions.
Running http://my-synology-ip/stats/?forexport exports everyting, while http://my-synology-ip/stats/export.json gets the result much quicker.
If needed, the url http://my-synology-ip/stats/index.php can be called to get the latest amount of pictures, without having to wait for the calculation fo the directory size.
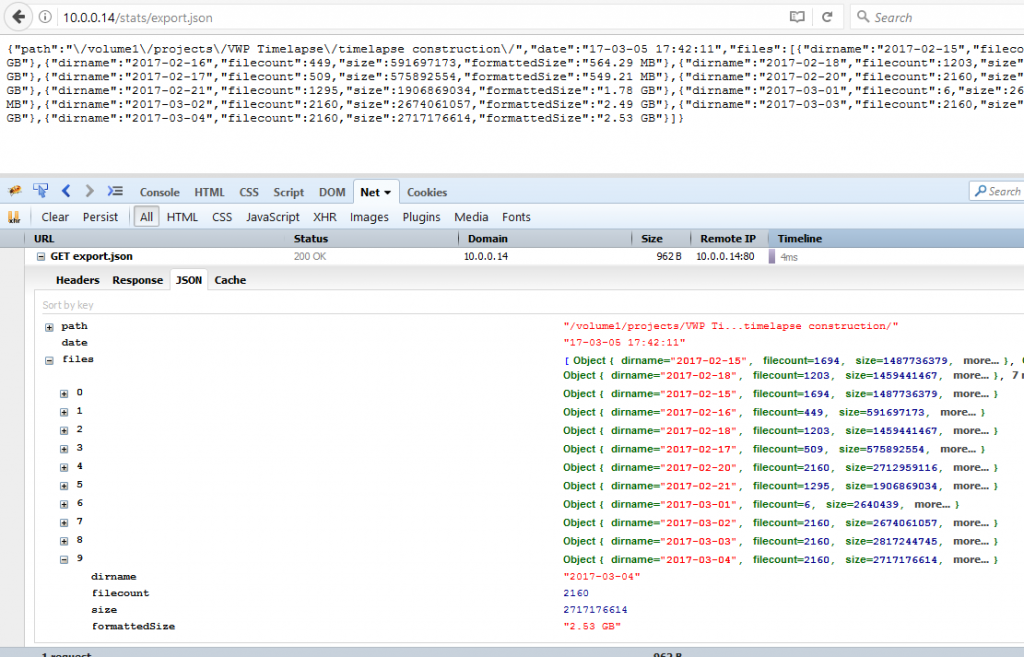