I do hate this, scrollbars and borders around my webpage.
As I have a webpage that is adding every post at the bottom, the height is dynamic, i.e. is not every time an x amount of pixels.
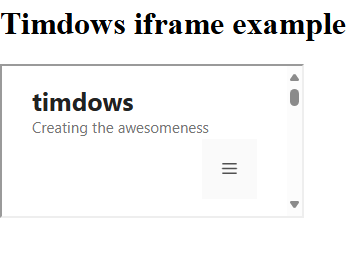
<!DOCTYPE html> <html> <body> <h1>Timdows iframe example</h1> <iframe src="https://timdows.com"> </iframe> </body> </html>
In order to remove some things, I turned myself into google. There are doznes of solutions, some work, some do not.
I want a clean vanilla javascript implementation (that I also understand and can maintain).
Lets setup the wordpress website that will host our iframe. In this case I use the plugin Elementor.
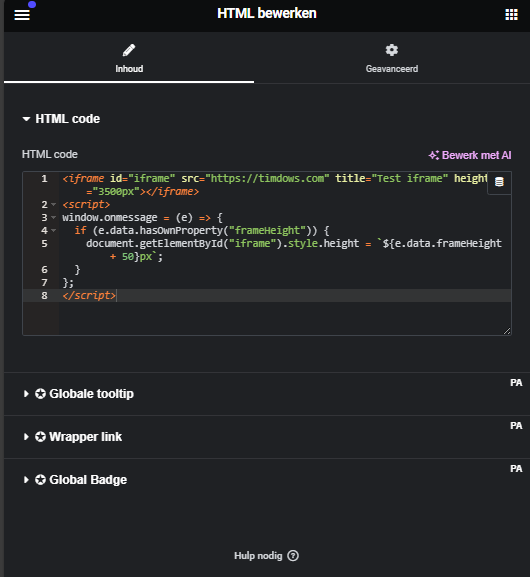
Hmm, lets see. I start with a height of 3500, that is way more than needed. Upon a message received, some javascript code is executed.
This code checks if there is a property called ‘frameHeight’ and gets the value of it, that is then (added with a nice 50px) set to the iframe element.
What happens is that the 3500pixel iframe is slunk to the correct height.
<iframe id="iframe" src="https://timdows.com" title="Test iframe" height="3500px"></iframe> <script> window.onmessage = (e) => { if (e.data.hasOwnProperty("frameHeight")) { document.getElementById("iframe").style.height = `${e.data.frameHeight + 50}px`; } }; </script>
This frameHeight is get from some angular methods. Lets dive into them.
– onInit, get the data from the service, and when it is received, use a 0 timeout in order to have the data bound to the DOM, then call sendPostMessage
– afterViewInit, make a listener if the window is resized, and then also call sendPostMessage
– sendPostMessage, it looks for the iframe-container (this is the most outer container of my page) and gets the container height. It uses postMessage to send data. In the body it sets the frameHeight property
<script> constructor( private pagesService: any, private el: ElementRef, private renderer: Renderer2) { } ngOnInit(): void { this.pagesService.getAllPages() .subscribe(data => { this.pageData = data; setTimeout(() => { this.sendPostMessage(); }, 0); }); } ngAfterViewInit(): void { this.renderer.listen('window', 'resize', () => this.sendPostMessage()); } private sendPostMessage(): void { const container = this.el.nativeElement.querySelector('#iframe-container'); if (this.height !== container.offsetHeight) { this.height = container.offsetHeight; window.parent.postMessage({ frameHeight: this.height }, '*'); console.log("The iframe-container frame height is:", this.height); } } </script>
After all of this, my page has no scrollbars anymore, and regardsless of the amount of content presented, it always sizes to the correct height.